Spring Security with Spring Doc Configuration
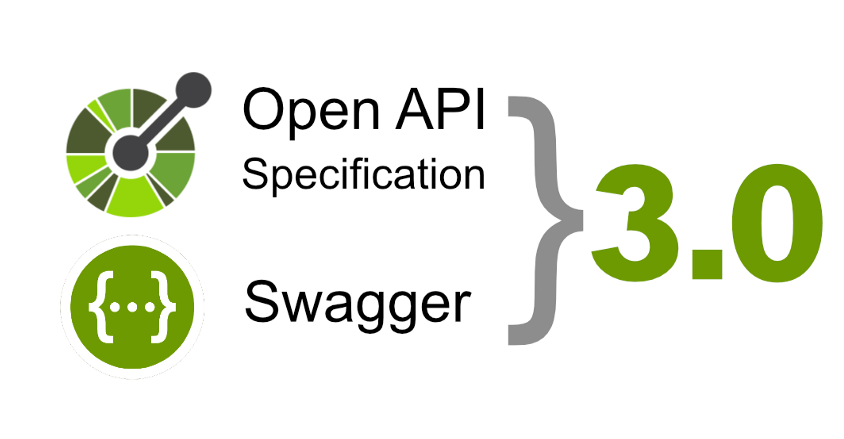
When building a Spring Boot application, you often need to secure your endpoints using Spring Security. At the same time, tools like Swagger UI or Springdoc OpenAPI help document and test your APIs. However, configuring Spring Security to allow access to Swagger resources while keeping your application secure can be tricky. This blog post outlines best practices and common configurations to ensure a smooth integration. ๐
Understanding the Challenge
Swagger UI and Springdoc OpenAPI rely on specific endpoints to serve API documentation and interactive UI. Typically, these endpoints include:
/swagger-ui/**
or/swagger-ui.html
/v3/api-docs/**
If these paths are not explicitly permitted in your Spring Security configuration, access to Swagger resources will be blocked by default. โ ๏ธ
To resolve this, you must update your SecurityFilterChain
configuration to whitelist these endpoints. โ
Basic Spring Security Configuration for Swagger
Here is a simple example of how to configure Spring Security to allow access to Swagger resources while securing other parts of your application:
@Configuration
@EnableWebSecurity
public class SecurityConfiguration {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.csrf(AbstractHttpConfigurer::disable) // Disable CSRF for simplicity
.authorizeHttpRequests(authz -> authz
.requestMatchers("/swagger-ui/**", "/v3/api-docs/**", "/swagger-ui.html").permitAll()
.anyRequest().authenticated()
);
return http.build();
}
}
Key Points:
.csrf(AbstractHttpConfigurer::disable)
: CSRF protection is often disabled for simplicity when using Swagger. However, you may want to secure it appropriately in production. ๐.requestMatchers
: These matchers specify the paths to allow unauthenticated access for Swagger resources..anyRequest().authenticated()
: Ensures that all other endpoints are secured. โ
Avoiding Interference with Custom Error Handling
If you use custom error handlers, such as overriding ErrorController
or implementing AuthenticationEntryPoint
, you might unintentionally block or alter Swagger-related responses. To avoid this, you can explicitly bypass your custom logic for Swagger paths. ๐ ๏ธ
Hereโs an example:
private boolean isSwaggerRequest(HttpServletRequest request) {
String uri = request.getRequestURI();
return uri.startsWith("/swagger-ui") || uri.startsWith("/v3/api-docs") || uri.startsWith("/swagger-ui.html");
}
@Override
public void commence(HttpServletRequest request, HttpServletResponse response,
AuthenticationException authException) throws IOException {
if (isSwaggerRequest(request)) {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Unauthorized");
return;
}
// Custom logic for other requests
}
Why This Matters
By explicitly bypassing Swagger-related requests in custom error handling, you ensure that these tools operate correctly without being affected by unrelated application-specific logic. ๐ง
Configuring CORS for Swagger
Swagger UI often makes AJAX calls to fetch API documentation. If youโre testing your application from a different origin (e.g., localhost vs. deployed server), youโll need to configure Cross-Origin Resource Sharing (CORS). ๐
Hereโs how you can configure CORS for Swagger:
@Bean
public CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(List.of("*")); // Allow all origins (adjust as needed)
configuration.setAllowedMethods(List.of("GET", "POST", "PUT", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(List.of("Authorization", "Content-Type", "Accept"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
Notes:
- Replace
*
with specific origins in production for enhanced security. ๐ - Swaggerโs AJAX requests will work seamlessly with properly configured CORS.
Testing the Configuration
To verify the setup:
- Start your Spring Boot application.
- Navigate to
http://localhost:8080/swagger-ui.html
orhttp://localhost:8080/swagger-ui/
. - Confirm that you can access Swagger UI without authentication. ๐
If you encounter issues, check:
- Whether the paths match the actual Swagger endpoints in your application.
- Your Spring Security configuration for potential misconfigurations.
- CORS settings if Swagger UI fails to fetch API docs. ๐ ๏ธ
Conclusion
Integrating Spring Security with Swagger and Springdoc OpenAPI requires careful configuration. By properly configuring SecurityFilterChain
, handling custom error logic, and setting up CORS, you can ensure that your API documentation is easily accessible while maintaining robust security for other endpoints. Following these best practices simplifies development and improves the overall developer experience. ๐